Previously we have done a project on “IoT motion sensor” using ESP8266 and IFTTT services but because now IFTTT has changed its plans now it is not a good option for us. So today we are going to do a similar project using ESP32 and telegram bot.
How does it work?
We will be using the telegram bot library in ESP32 to communicate with our telegram bot and whenever there is motion, the ESP32 will detect it using the sensor connected to the GPIO pins of the ESP32. Then an alert will be sent to the telegram bot and we will get a notification on our mobile device.
Follow the steps below to make one…
Hardware Required
For this example, we are using an IR obstacle sensor to detect the motion. Based on your purpose you can use any sensors like PIR, Microwave, Ultrasonic, etc… to detect the motion. Maybe there will be small changes in the code and circuit but the basics are the same. If you have any doubts ask me in the comments below.
Libraries Used
Download and install the above two libraries from the above GitHub link provided or install them via the Library Manager in the Arduino IDE. If you do not know how to it the follow the below steps,
Telegram Bot Library
To establish communication with the Telegram bot, we’ll be using the Universal Telegram Bot Library created by Brian Lough that provides an easy interface for Telegram Bot API.
Follow the next steps to install the latest release of the library.
1. Click this link to download the Universal Arduino Telegram Bot library.
2. Go to Sketch > Include Library > Add.ZIP Library...
3. Add the library you’ve just downloaded. And that’s it. The library is installed.
For details about the library, you can check out the Universal Arduino Telegram Bot Library GitHub page.
ArduinoJson Library
We also have to install the ArduinoJson library. Follow the next steps to install the library.
1. Go to Sketch > Include Library > Manage Libraries.
2. Search for “ArduinoJson”.
3. Select the latest version available.
4. Install the library.
Note
The versions of libraries used while making this tutorial is,
ArduinoJSON – 6.18.5
Telegram Bot – 1.3.0
Circuit
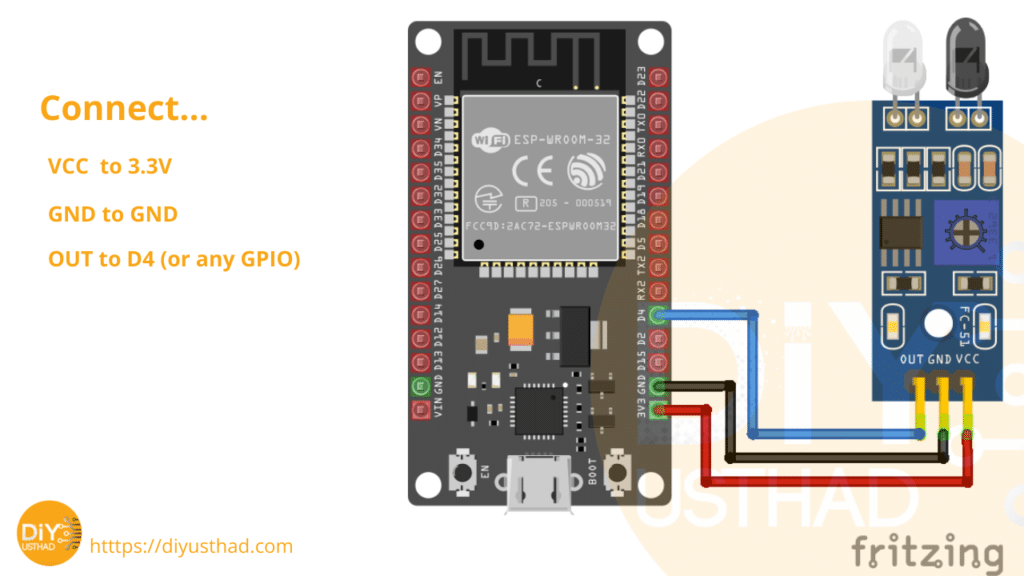
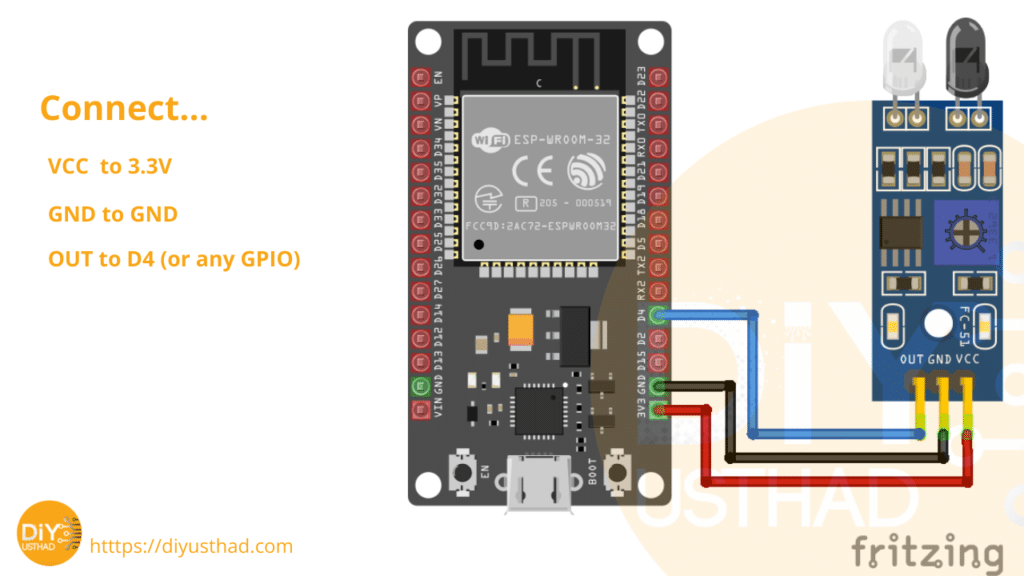
- Connect the VCC of the IR obstacle sensor to the 3.3V of the ESP32 devlopment board.
- Connect the GND of IR sensor to the GND of ESP32.
- Finally connect the OUT pin of the IR obstacle sensor to the GPIO D4 of ESP32 NodeMCU board or which ever ESP32 development board you are using.
Telegram bot creation
We need to create a telegram bot so that we can receive the “motion detected” notifications from the ESP32. Follow the below guide for creating a telegram bot.
After creating the bot get the bot token and chat ID and paste it in the below Arduino code where it is mentioned.
Code
#include <WiFi.h> #include <WiFiClientSecure.h> #include <UniversalTelegramBot.h> // Wifi network station credentials #define WIFI_SSID "YOUR SSID" #define WIFI_PASSWORD "YOUR WIFI PASSWORD" // Telegram BOT Token (Get from Botfather) #define BOT_TOKEN "YOUR BOT TOKEN" #define chat_id "YOUR CHAT ID" WiFiClientSecure secured_client; UniversalTelegramBot bot(BOT_TOKEN, secured_client); const int sensor = 4; int flag = 0; void setup() { Serial.begin(115200); Serial.println(); pinMode(sensor, INPUT); digitalWrite(sensor, HIGH); // attempt to connect to Wifi network: Serial.print("Connecting to Wifi SSID "); Serial.print(WIFI_SSID); WiFi.begin(WIFI_SSID, WIFI_PASSWORD); secured_client.setCACert(TELEGRAM_CERTIFICATE_ROOT); // Add root certificate for api.telegram.org while (WiFi.status() != WL_CONNECTED) { Serial.print("."); delay(500); } Serial.print("\nWiFi connected. IP address: "); Serial.println(WiFi.localIP()); } void loop() { boolean sensorState = digitalRead(sensor); if (sensorState == LOW && flag == 0) { bot.sendMessage(chat_id, "Obstacle Detected", ""); flag = 1; } else if (sensorState == HIGH && flag == 1) { bot.sendMessage(chat_id, "Obstacle Removed", ""); flag = 0; } }
Some commercial IoT motion sensors
Affiliate links
Applications
- IoT motion sensor
- IoT obstacle detector (as we have done here)
- IoT gas leak detector.
- IoT weather station.
Conclusion
So in this project, we have learned to send sensor data to a telegram bot from ESP32 and we have used that knowledge to make an IoT motion sensor/ obstacle detector. As I mentioned above, we can use any other sensors based on our application and send their data to our telegram bot.
im using the pir sensor so can you tell me what change in the coding