In this tutorial, we are going to build a simple OTG Phone Thermometer using Digispark ATtiny85 that can connect to our mobile phone via an OTG adaptor and read the real-time temperature of anything and display it on the serial monitor.
How it works!
The Digispark ATtiny85 will connect to the phone using the DigiCDC library which allows the Digispark to appear to a mobile or computer as a Virtual Serial Port when connected by USB. This makes it appear just like a standard Arduino and allows the use of the Serial Monitor. Then we read the temperature using a Precision thermistor and we use the Serial print function to print it on the serial monitor.


Things Required
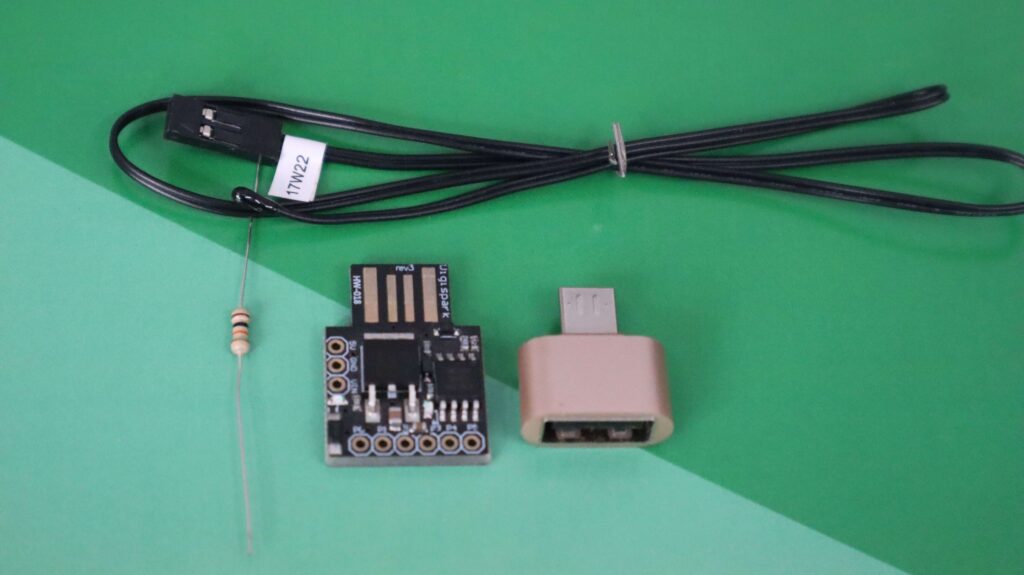
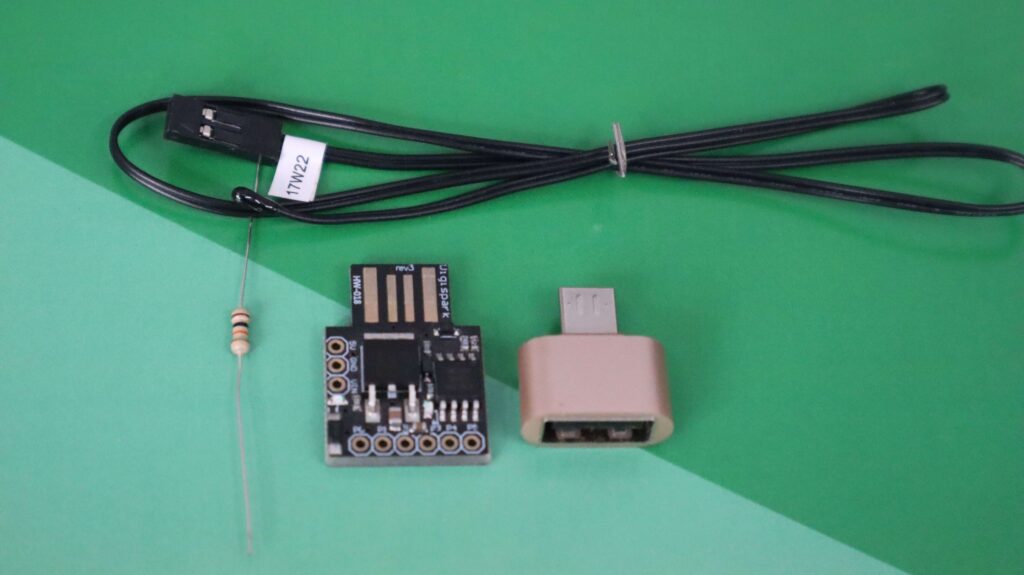
Software Required
Install the Serial USB Terminal application from the google play store. This app will help us read and display the serial data from the Digispark Attiny85 board.
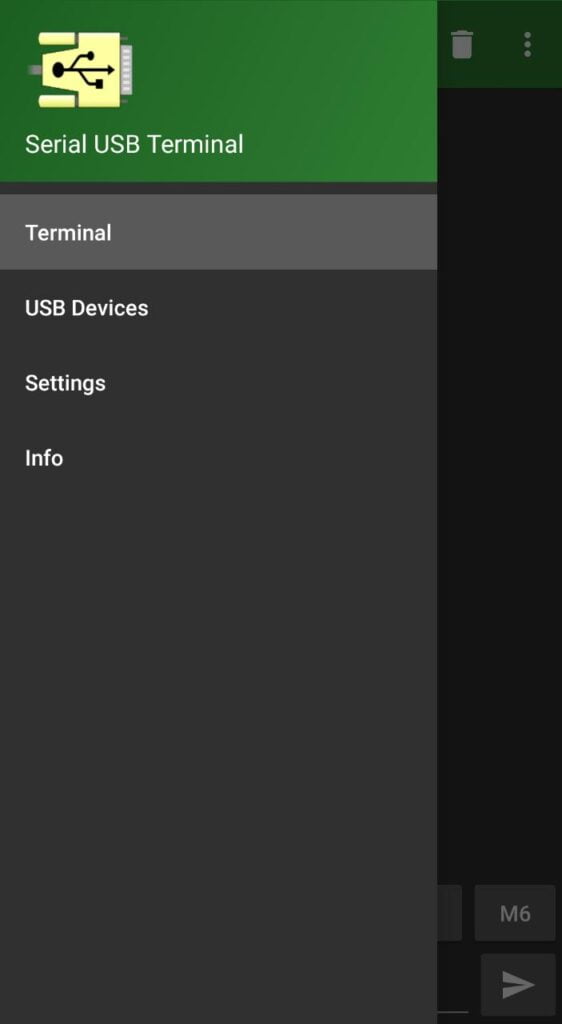
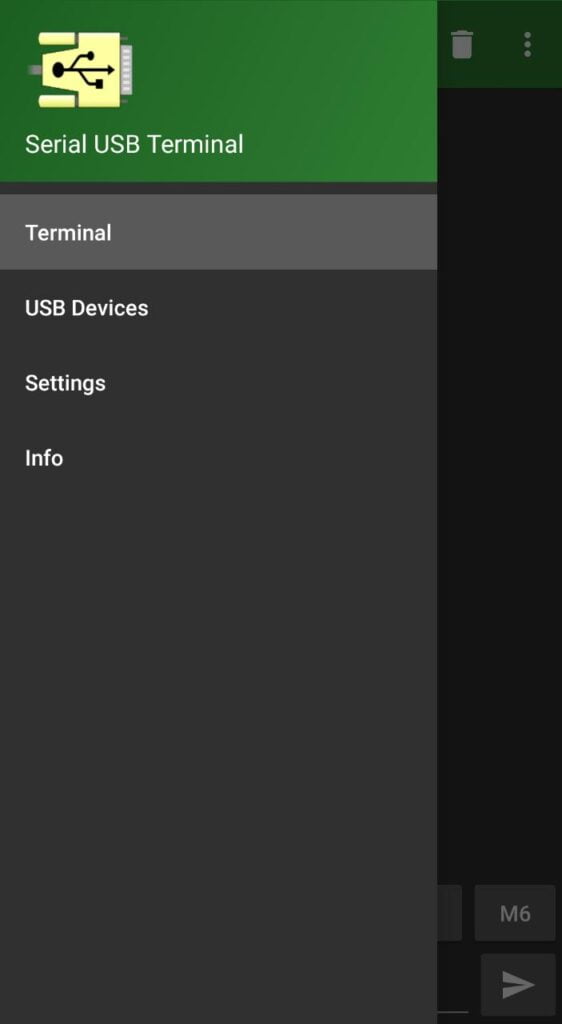
Circuit
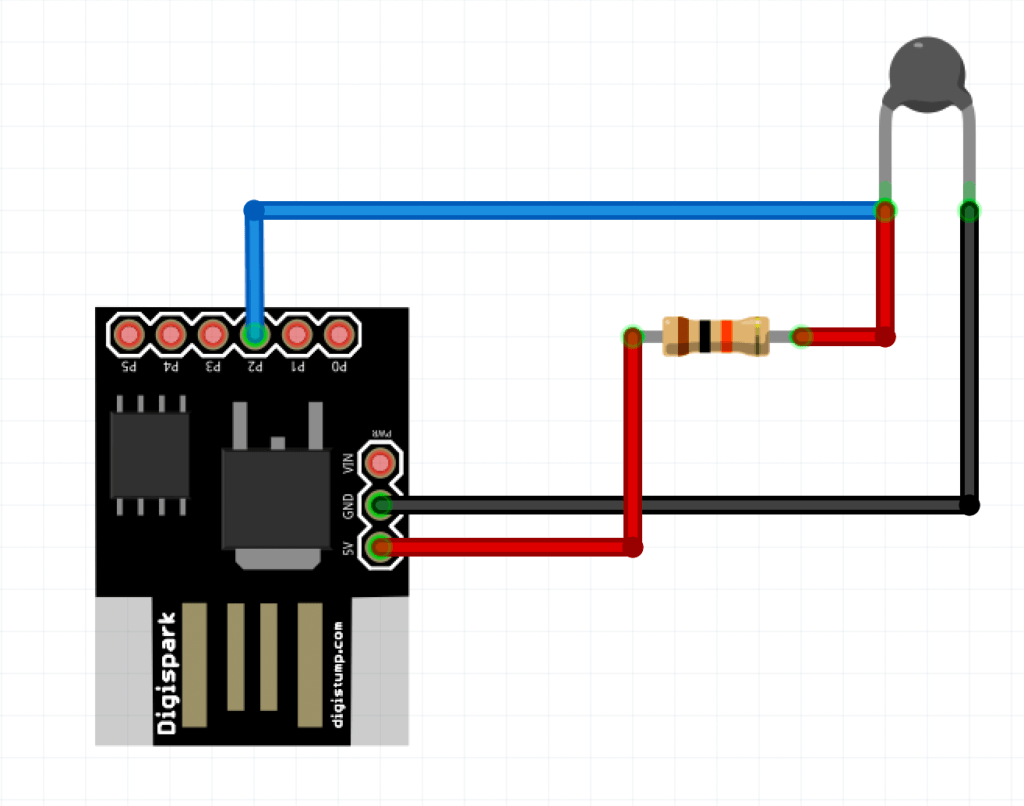
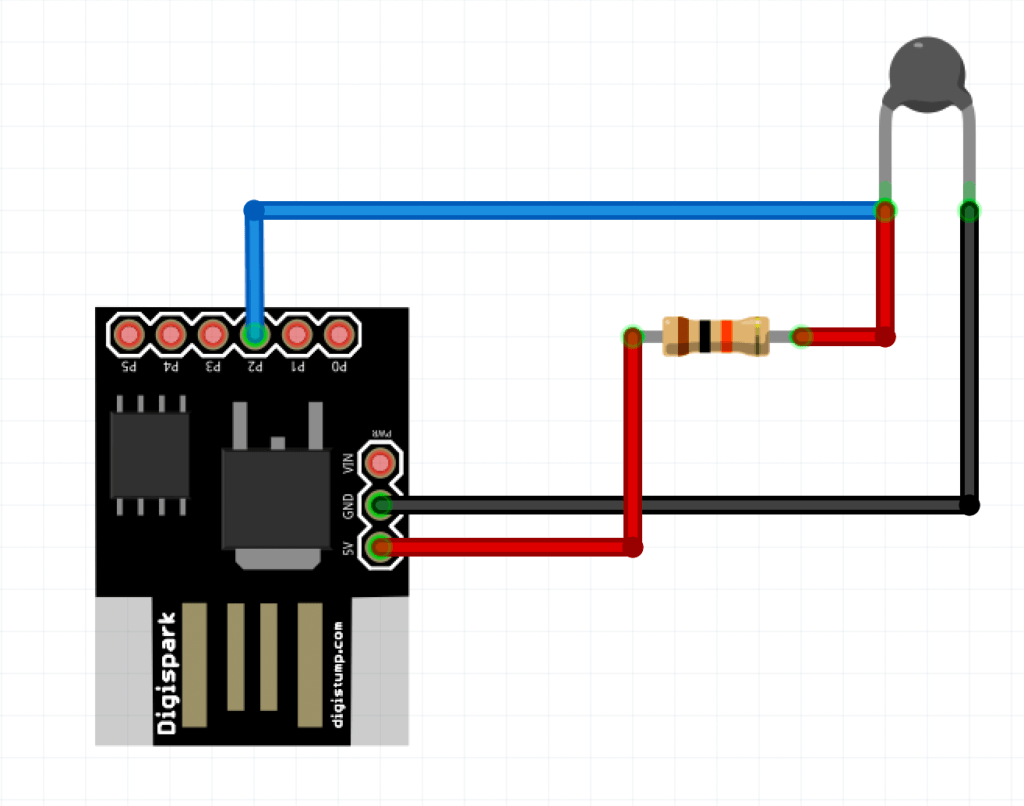
The connections are very straight forward, follow the below steps.
- Connect one terminal of the thermistor to PIN P2 of Digispark ATtiny85.
- Connect the other terminal of the thermistor to the GND.
- Then connect a 10K resistor between the PIN P2 and 5V.
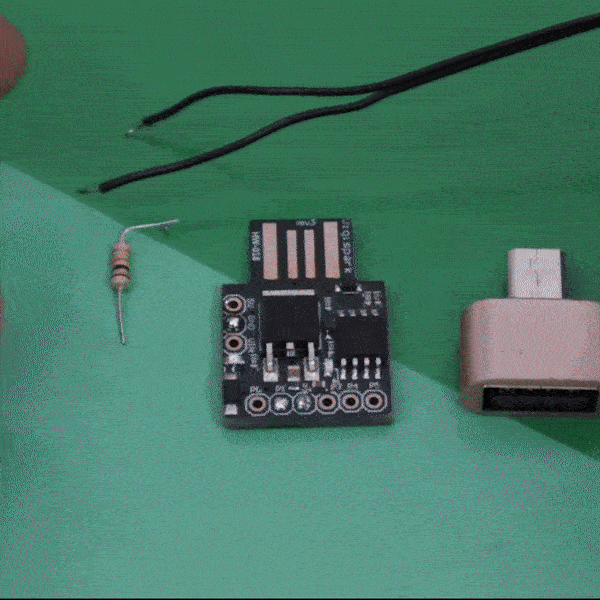
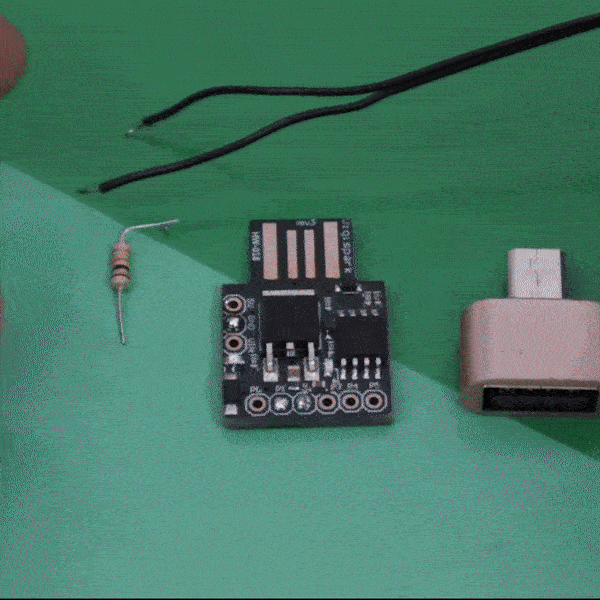
Code
Upload the provided code to Digispark attiny85 using the Arduino IDE.
For more info on using Digispark attiny85 with Arduino IDE follow this tutorial
https://www.hackster.io/alaspuresujay/use-an-attiny85-with-arduino-ide-07740c
#include <DigiCDC.h> // the value of the 'other' resistor #define SERIESRESISTOR 10000 // What pin to connect the sensor to #define THERMISTORPIN A1 #define THERMISTORNOMINAL 10000 // temp. for nominal resistance (almost always 25 C) #define TEMPERATURENOMINAL 25 // how many samples to take and average, more takes longer // but is more 'smooth' #define NUMSAMPLES 5 // The beta coefficient of the thermistor (usually 3000-4000) #define BCOEFFICIENT 3950 void setup() { SerialUSB.begin(); } // the loop routine runs over and over again forever: void loop() { if (SerialUSB.available()) { uint8_t i; float average; average = analogRead(THERMISTORPIN); // convert the value to resistance average = 1023 / average - 1; average = SERIESRESISTOR / average; //SerialUSB.print("Thermistor resistance "); //SerialUSB.println(average); float steinhart; float presteinhart; steinhart = average / THERMISTORNOMINAL; // (R/Ro) steinhart = log(steinhart); // ln(R/Ro) steinhart /= BCOEFFICIENT; // 1/B * ln(R/Ro) steinhart += 1.0 / (TEMPERATURENOMINAL + 273.15); // + (1/To) steinhart = 1.0 / steinhart; // Invert steinhart -= 273.15; // convert absolute temp to C if (int(presteinhart) != int(steinhart)) { SerialUSB.print("Temperature "); SerialUSB.print(steinhart); SerialUSB.println(" *C"); presteinhart = steinhart; } SerialUSB.delay(1000); } }
In Action!!!
Now it time to test our phone thermometer, follow the below steps carefully.
- Open the serial USB terminal app.
- Click the connect icon, when prompted to give access click OK
- To start receiving the temperature values we need to send some string, so just type something and click send.
- That’s it now we can read the real-time temperature of any objects from our phone.
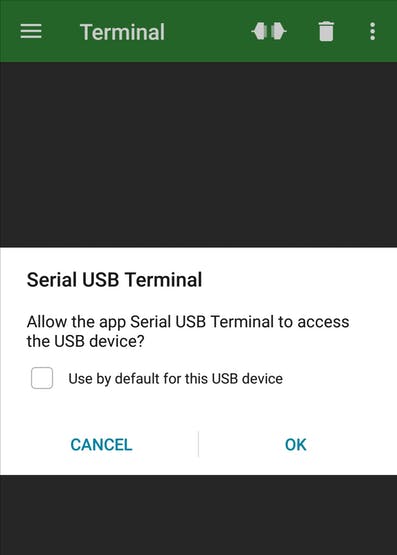
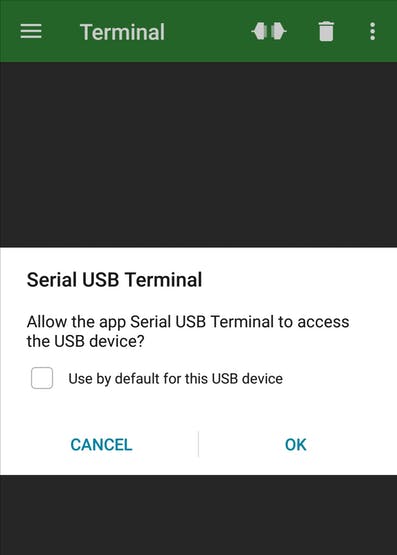
In the below video I’m checking the temperature of a cup of tea. ENJOY!