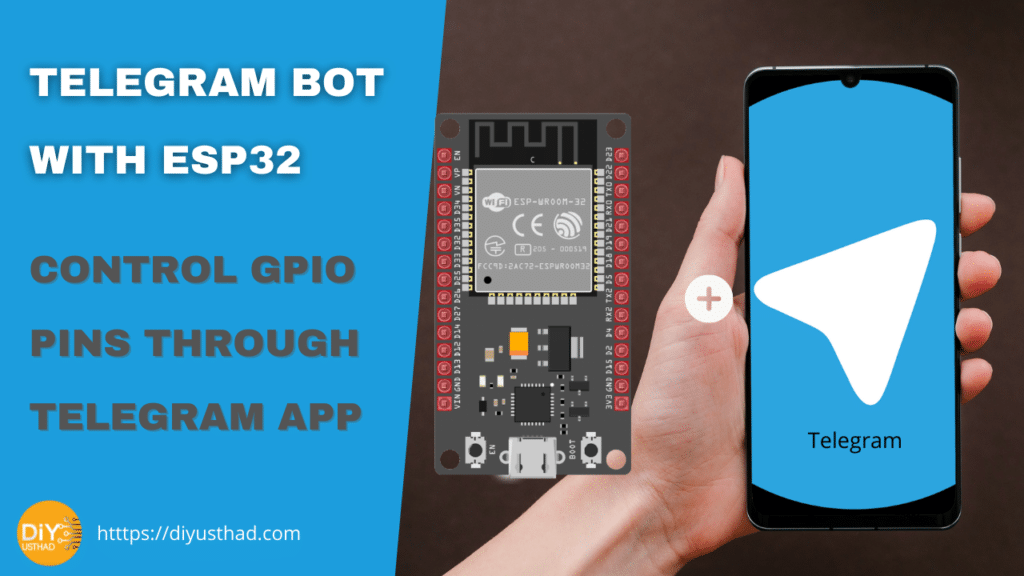
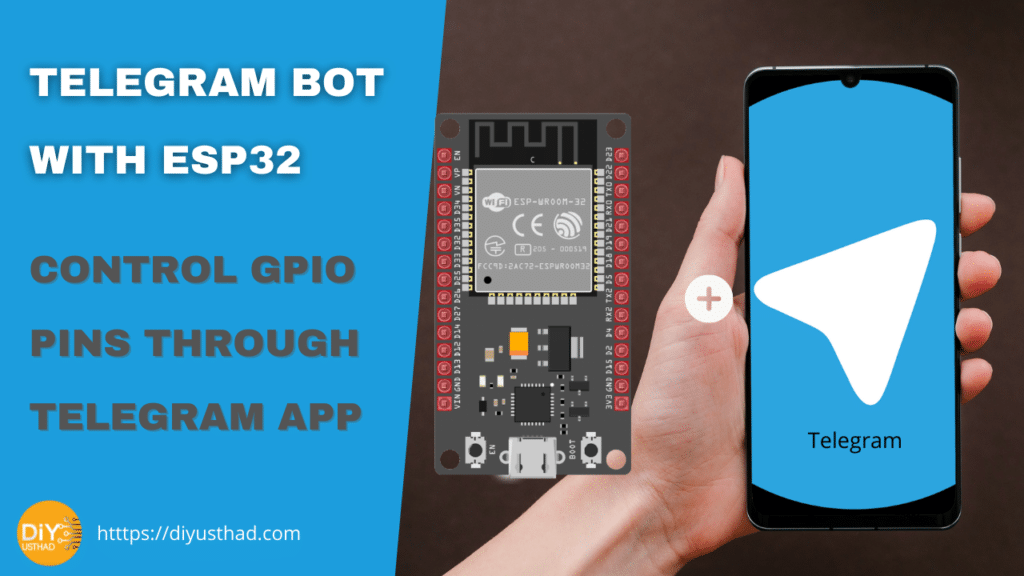
In this tutorial, we are going to learn about the telegram bot and how to use it with an ESP32/8266 board. By using telegram bot API in the ESP32/8266 we can control the GPIO pins or we can read the sensor data and send it to our mobile devices via the telegram messengers.
The telegram messenger is free and because everything is working through the internet we can control and receive information from the ESP32 or ESP8266 boards where ever we are in the world. Using Telegram with ESP can make great IoT projects.
What is Telegram Bot
Bots are third-party applications that run inside Telegram. Users can interact with bots by sending them messages, commands, and inline requests. We can control our bots using HTTPS requests to Telegram Bot API.
Read mode on telegram bots from their official documentation https://core.telegram.org/bots
How to create a Telegram Bot
- Install Telegram from play store or apple store.
- make an account if you don’t have one already.
- Then, search for “botfather” and click the BotFather as shown in the video. Botfather is a pre-built Telegram bot that lets you create, manage, and delete your bots.
- Click on the start button and choose /newbot
- Give your bot a name and username.
- If your bot is successfully created, you’ll receive a message with a link to access your new bot and the bot token. Bot token is a unique id that we to communicate with the bot.
Get your Telegram chat id
In the telegram messenger every user, chat, and group is having a unique ID. So To prevent any unauthorized access to our bots from other users we need to find our unique user ID and use it inside our Arduino code for ESP32.
By doing so, every time ESP receives a message from the bot, it checks whether the ID corresponds with the ID pre-defined in the code, and then only if it matches it executes the commands. Follow the below steps to find your Used ID.
- In your Telegram account, search for “@myidbot” or open this link t.me/myidbot on your smartphone.
- Start a conversation with that bot and type /getid. You will get a reply back with your user ID.
- Note the user id as we’ll need it later.
Hardware Used
Libraries Used in Arduino IDE
We will be using Arduino IDE for programming the ESP32 board. So, make sure you have the IDE as well as the board package installed on your computer. If you have not set up the Arduino IDE to use with the ESP32 board then follow this tutorial here
We need to install the below two libraries in Arduino IDE, we need these two libraries for communicating with the telegram bot.
Telegram Bot Library
To establish communication with the Telegram bot, we’ll be using the Universal Telegram Bot Library created by Brian Lough that provides an easy interface for Telegram Bot API.
Follow the next steps to install the latest release of the library.
1. Click this link to download the Universal Arduino Telegram Bot library.
2. Go to Sketch > Include Library > Add.ZIP Library...
3. Add the library you’ve just downloaded. And that’s it. The library is installed.
For details about the library, you can check out the Universal Arduino Telegram Bot Library GitHub page.
ArduinoJson Library
We also have to install the ArduinoJson library. Follow the next steps to install the library.
1. Go to Sketch > Include Library > Manage Libraries.
2. Search for “ArduinoJson”.
3. Select the latest version available.
4. Install the library.
Note
The versions of libraries used while making this tutorial is,
ArduinoJSON – 6.18.5
Telegram Bot – 1.3.0
Programming the ESP32
The below code is taken from the example program from the above-mentioned telegram library and I have made some minor changes.
Before uploading the code to your board you have to add your WIFI SSID, WIFI Password, Bot Token, and User ID in the mentioned positions in the code.
Code
#include <WiFi.h> #include <WiFiClientSecure.h> #include <UniversalTelegramBot.h> // Wifi network station credentials #define WIFI_SSID "YOUR WIFI SSID" #define WIFI_PASSWORD "YOUR WIFI PASSWORD" // Telegram BOT Token (Get from Botfather) #define BOT_TOKEN "YOUR BOT TOKEN" #define CHAT_ID "YOUR CHAT ID" const unsigned long BOT_MTBS = 1000; // mean time between scan messages WiFiClientSecure secured_client; UniversalTelegramBot bot(BOT_TOKEN, secured_client); unsigned long bot_lasttime; // last time messages' scan has been done const int ledPin = LED_BUILTIN; int ledStatus = 0; void handleNewMessages(int numNewMessages) { Serial.print("handleNewMessages "); Serial.println(numNewMessages); for (int i = 0; i < numNewMessages; i++) { String chat_id = String(bot.messages[i].chat_id); if (chat_id != CHAT_ID ) { bot.sendMessage(chat_id, "Unauthorized user", ""); } else { String text = bot.messages[i].text; String from_name = bot.messages[i].from_name; if (from_name == "") from_name = "Guest"; if (text == "/ledon") { digitalWrite(ledPin, HIGH); // turn the LED on (HIGH is the voltage level) ledStatus = 1; bot.sendMessage(chat_id, "Led is ON", ""); } if (text == "/ledoff") { ledStatus = 0; digitalWrite(ledPin, LOW); // turn the LED off (LOW is the voltage level) bot.sendMessage(chat_id, "Led is OFF", ""); } if (text == "/status") { if (ledStatus) { bot.sendMessage(chat_id, "Led is ON", ""); } else { bot.sendMessage(chat_id, "Led is OFF", ""); } } if (text == "/start") { String welcome = "Welcome to Universal Arduino Telegram Bot library, " + from_name + ".\n"; welcome += "This is Flash Led Bot example.\n\n"; welcome += "/ledon : to switch the Led ON\n"; welcome += "/ledoff : to switch the Led OFF\n"; welcome += "/status : Returns current status of LED\n"; bot.sendMessage(chat_id, welcome, "Markdown"); } } } } void setup() { Serial.begin(115200); Serial.println(); pinMode(ledPin, OUTPUT); // initialize digital ledPin as an output. delay(10); digitalWrite(ledPin, LOW); // initialize pin as off (active LOW) // attempt to connect to Wifi network: Serial.print("Connecting to Wifi SSID "); Serial.print(WIFI_SSID); WiFi.begin(WIFI_SSID, WIFI_PASSWORD); secured_client.setCACert(TELEGRAM_CERTIFICATE_ROOT); // Add root certificate for api.telegram.org while (WiFi.status() != WL_CONNECTED) { Serial.print("."); delay(500); } Serial.print("\nWiFi connected. IP address: "); Serial.println(WiFi.localIP()); } void loop() { if (millis() - bot_lasttime > BOT_MTBS) { int numNewMessages = bot.getUpdates(bot.last_message_received + 1); while (numNewMessages) { Serial.println("got response"); handleNewMessages(numNewMessages); numNewMessages = bot.getUpdates(bot.last_message_received + 1); } bot_lasttime = millis(); } }
Testing
After burning the code to your ESP32 board, open the serial monitor to check whether the ESP is connecting to the internet. don’t forget to set the baud rate to 115200 in the serial monitor. If the ESP is successfully connected to the internet then the IP address will be printed.
Now open the Telegram app on your mobile device then type and send /start to your bot and it should return some information about how to turn ON, OFF, Status of the LED. All these messages we receive are coming from the Arduino code.
You can use /ledon for turning ON the LED, /ledoff for turn OFF, and /status for check the status of the LED, if you want to change that you can change it in the Arduino code.
Conclusion
So we are successfully learned how to use the Telegram bot with the ESP32 board and to control the inbuilt LED from our mobile device via the Telegram messenger app. Like the same, you can control any GPIO pins on the ESP and even make home automation projects by connecting some relays to your ESP development board.
In our next tutorial inSha’Allah we are going to learn how to read some sensor values like temperature or humidity and send them periodically to our mobile device. So stay tune guys. If you have not yet subscribed to our newsletter, you can subscribe from the form below this page so you will receive important updates weekly.