In this project, we are going to make an Android app with flutter which will communicate with Arduino via USB serial to read the data from the HC-SR04 ultrasonic module and to display the output as a measurement in centimeters.
Concept
The Arduino will take the raw data from the HC-SR04 ultrasonic module and convert it into measurement in centimeters and transmit the data to our android phone via the USB OTG.
Then our flutter app will receive the information from the Arduino and display it in radial gauge as you can see in the image here.
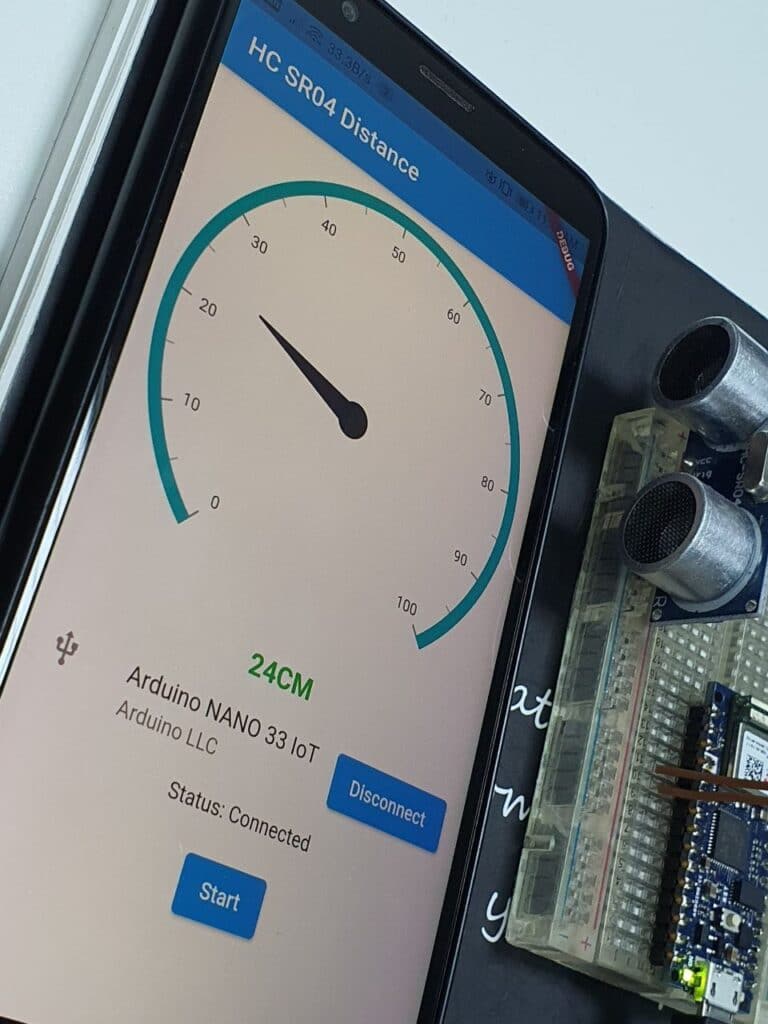
Notice
To continue with this project You should have some basic knowledge in dart programming language and flutter.
Components Used
Components | AliExpress![]() ![]() | Amazon![]() ![]() | Banggood![]() ![]() |
---|---|---|---|
Arduino Nano (in this project I have used nano 33 but old nano will also work) | ![]() ![]() | ![]() ![]() | ![]() ![]() |
Bread Board | ![]() ![]() | ![]() ![]() | ![]() ![]() |
Jumper Wires | ![]() ![]() | ![]() ![]() | ![]() ![]() |
HC-SR04 Ultrasonic Module | ![]() ![]() | ![]() ![]() | ![]() ![]() |
Circuit
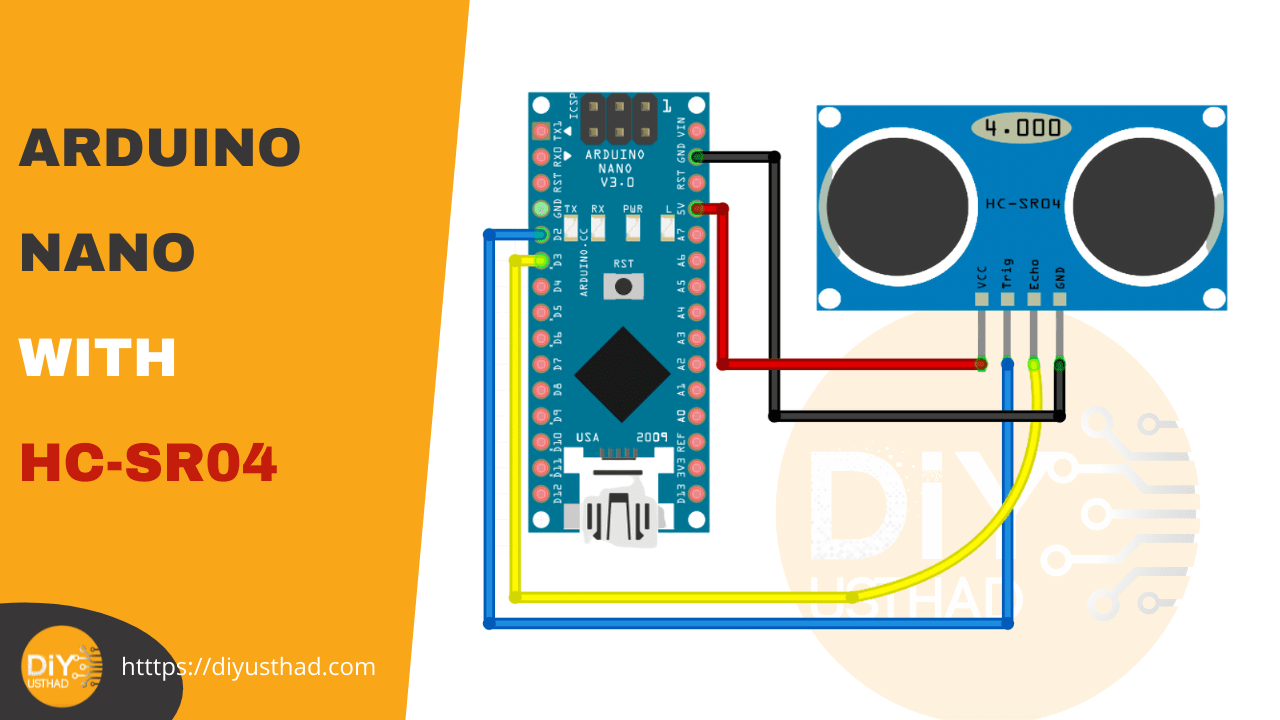
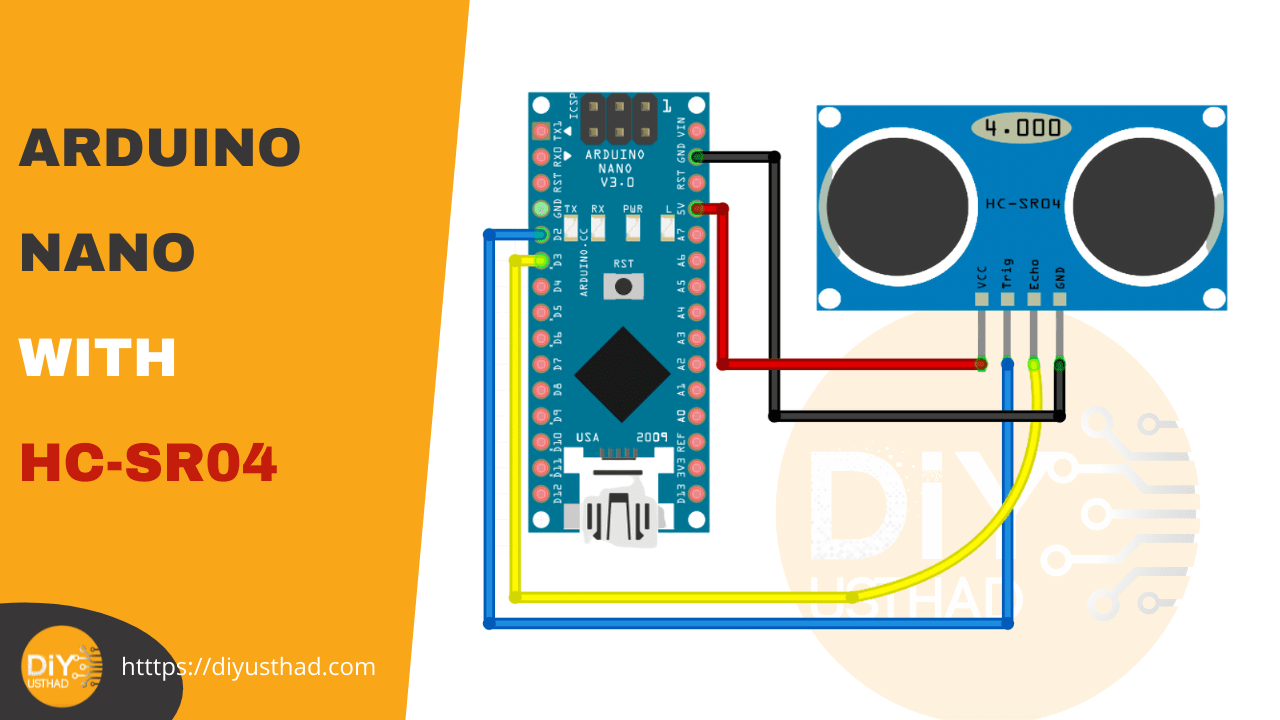
Connect the Arduino and HC-SR04 module as per the above circuit diagram, you can use any digital pins of Arduino for the echo and trigger pin but make sure it’s the same as both in the circuit and the code.
Arduino | HC- SR04 |
---|---|
5V | Vcc |
Digital pin 2 | Trig ( Trigger Pulse Input ) |
Digital pin 3 | Echo( Echo Pulse Output ) |
GND | GND |
Arduino Code
Upload the below code to the Arduino nano, the code is very basic it just read the data from the HC-SR04 ultrasonic module and convert it to centimeter and print it to the serial.
const int trig = 2, echo = 3; long duration, Distanceincm; void setup() { Serial.begin(115200); pinMode(trig, OUTPUT); pinMode(echo, INPUT); } void loop() { digitalWrite(trig, HIGH); delay(15); digitalWrite(trig, LOW); duration = pulseIn(echo, HIGH); Distanceincm = duration / 58; Serial.println(Distanceincm); delay(500); }
Flutter App
The code and instructions for the flutter app are given below.
Packages Used
usb_serial 0.3.0 | https://pub.dev/packages/usb_serial |
syncfusion_flutter_gauges 19.2.51 | https://pub.dev/packages/syncfusion_flutter_gauges |
Add the above packages to the pubspec.yaml file as shown below. Note that the usb_serial 0.3.0 package will only support android, no support for iPhone.
dependencies: flutter: sdk: flutter syncfusion_flutter_gauges: ^19.2.51 usb_serial: ^0.3.0
Dart Code
import 'dart:async'; import 'dart:typed_data'; import 'package:flutter/material.dart'; import 'package:usb_serial/transaction.dart'; import 'package:usb_serial/usb_serial.dart'; import 'package:syncfusion_flutter_gauges/gauges.dart'; void main() => runApp(MyApp()); class MyApp extends StatefulWidget { @override _MyAppState createState() => _MyAppState(); } class _MyAppState extends State<MyApp> { UsbPort? _port; String _status = "Idle"; List<Widget> _ports = []; List<Widget> _serialData = []; double myValue = 0; StreamSubscription<String>? _subscription; Transaction<String>? _transaction; UsbDevice? _device; Future<bool> _connectTo(device) async { _serialData.clear(); if (_subscription != null) { _subscription!.cancel(); _subscription = null; } if (_transaction != null) { _transaction!.dispose(); _transaction = null; } if (_port != null) { _port!.close(); _port = null; } if (device == null) { _device = null; setState(() { _status = "Disconnected"; }); return true; } _port = await device.create(); if (await (_port!.open()) != true) { setState(() { _status = "Failed to open port"; }); return false; } _device = device; await _port!.setDTR(true); await _port!.setRTS(true); await _port!.setPortParameters( 115200, UsbPort.DATABITS_8, UsbPort.STOPBITS_1, UsbPort.PARITY_NONE); _transaction = Transaction.stringTerminated( _port!.inputStream as Stream<Uint8List>, Uint8List.fromList([13, 10])); _subscription = _transaction!.stream.listen((String line) { setState(() { _serialData.clear(); _serialData.add(Text( line + "CM", style: TextStyle( fontSize: 20, color: Colors.green, fontWeight: FontWeight.bold), )); myValue = double.parse(line); }); }); setState(() { _status = "Connected"; }); return true; } void _getPorts() async { _ports = []; List<UsbDevice> devices = await UsbSerial.listDevices(); if (!devices.contains(_device)) { _connectTo(null); } print(devices); devices.forEach((device) { _ports.add(ListTile( leading: Icon(Icons.usb), title: Text(device.productName!), subtitle: Text(device.manufacturerName!), trailing: ElevatedButton( child: Text(_device == device ? "Disconnect" : "Connect"), onPressed: () { _connectTo(_device == device ? null : device).then((res) { _getPorts(); }); }, ))); }); setState(() { print(_ports); }); } @override void initState() { super.initState(); UsbSerial.usbEventStream!.listen((UsbEvent event) { _getPorts(); }); _getPorts(); } @override void dispose() { super.dispose(); _connectTo(null); } @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar( title: const Text('HC SR04 Distance'), ), body: Center( child: Column( children: <Widget>[ SfRadialGauge( axes: <RadialAxis>[ RadialAxis( axisLineStyle: AxisLineStyle(color: Colors.blue), minimum: 0, maximum: 200, pointers: <GaugePointer>[ NeedlePointer(value: myValue), ], ), ], ), ..._serialData, ..._ports, Text('Status: $_status\n'), Card( child: Text( 'https://diyusthad.com', style: TextStyle(color: Colors.yellow.shade700), ), ), ], ), ), ), ); } }
Great work!