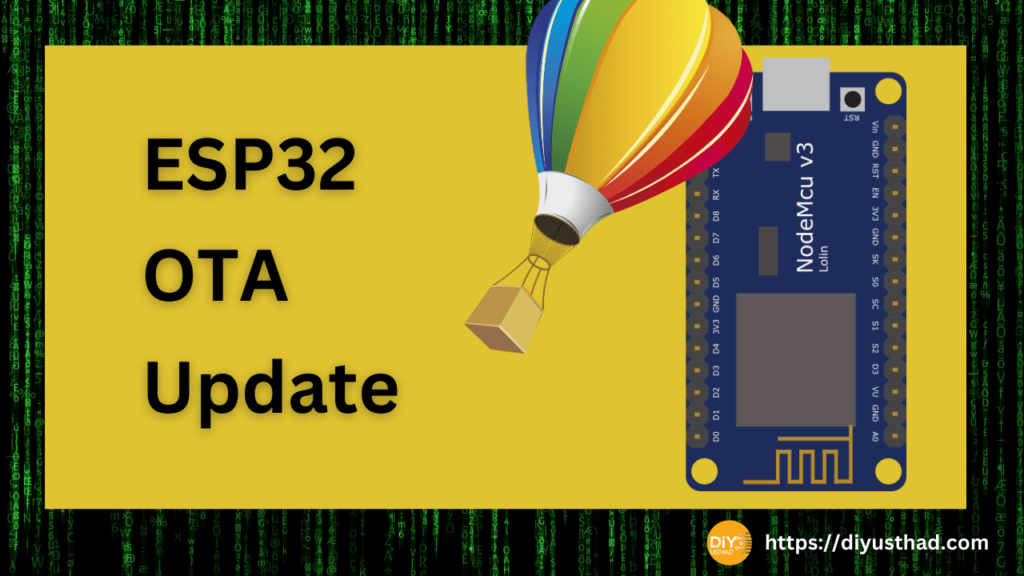
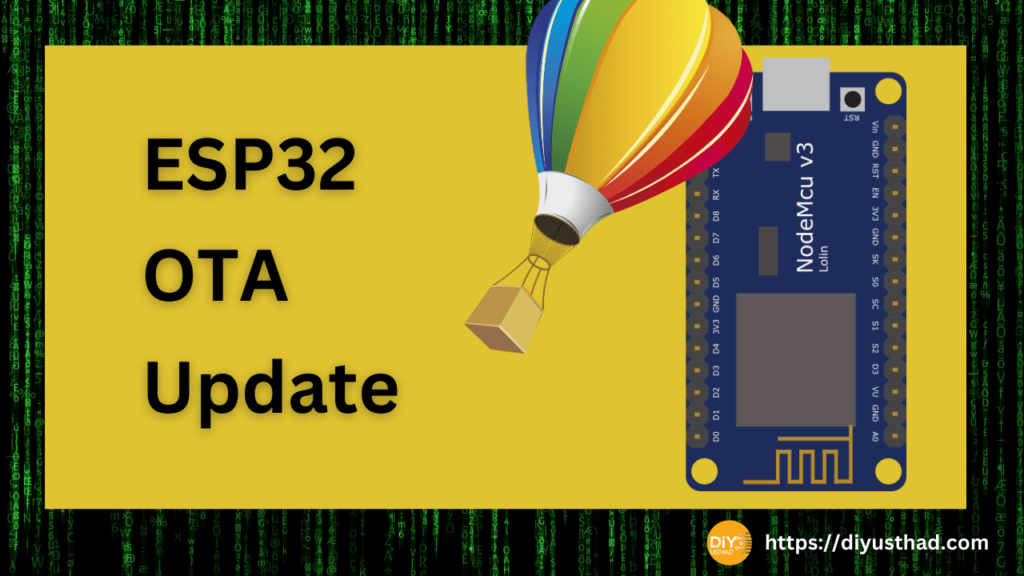
The ESP32 is a microcontroller that is capable of performing over-the-air (OTA) updates. OTA updates allow you to update the firmware of your ESP32 wirelessly, without the need to physically connect it to a computer.
What is OTA update?
Over-the-air (OTA) updates refer to the process of updating the firmware or software of a device wirelessly, without the need to physically connect it to a computer. OTA updates are useful because they allow you to update the device remotely, and they can save time and effort by eliminating the need to physically access the device to perform the update.
OTA updates are commonly used for devices that are installed in hard-to-reach locations or that need to be updated frequently, such as Internet of Things (IoT) devices.
Method 1: ArduinoOTA Library
To perform OTA updates, you will need to set up a web server that serves the firmware update file and configure the ESP32 to connect to this server and download the update file. You can then use the ArduinoOTA library to perform the update.
Example
Here is an example of code that demonstrates how to set up OTA updates on the ESP32 using the ArduinoOTA library:
- First, you will need to include the ArduinoOTA and WiFi libraries at the top of your sketch:
#include <ArduinoOTA.h> #include <WiFi.h>
- Next, set up the WiFi connection by calling the WiFi.begin() function and passing in your WiFi network name and password:
const char* ssid = "your_wifi_name"; const char* password = "your_wifi_password"; WiFi.begin(ssid, password); while (WiFi.status() != WL_CONNECTED) { delay(500); Serial.print("."); }
- Initialize the ArduinoOTA library by calling the ArduinoOTA.begin() function:
ArduinoOTA.begin();
- Set the hostname and password for the OTA update using the ArduinoOTA.setHostname() and ArduinoOTA.setPassword() functions:
ArduinoOTA.setHostname("esp32-ota"); ArduinoOTA.setPassword("password");
- Set up a function to handle incoming OTA update requests using the ArduinoOTA.onStart() function:
void handleOTAStart() { // Perform any necessary tasks before the update begins, such as saving data or closing file handles Serial.println("OTA update starting..."); } ArduinoOTA.onStart(handleOTAStart);
- Set up a function to handle the progress of the OTA update using the ArduinoOTA.onProgress() function:
void handleOTAProgress(unsigned int progress, unsigned int total) { // Display the progress of the update to the user Serial.printf("Progress: %u%%\r", (progress / (total / 100))); } ArduinoOTA.onProgress(handleOTAProgress);
- Set up a function to handle the completion of the OTA update using the ArduinoOTA.onEnd() function:
void handleOTAEnd() { // Perform any necessary tasks after the update is complete, such as restarting the ESP32 Serial.println("\nOTA update complete!"); ESP.restart(); } ArduinoOTA.onEnd(handleOTAEnd);
- Finally, call the ArduinoOTA.handle() function in the main loop of your program to listen for and handle OTA update requests:
void loop() { ArduinoOTA.handle(); // Your other code goes here }
That’s it! With these steps, you should now be able to perform OTA updates on your ESP32. Remember to test your OTA updates thoroughly before deploying them to production devices.
Full Code
#include <ArduinoOTA.h> #include <WiFi.h> const char* ssid = "your_wifi_name"; const char* password = "your_wifi_password"; void handleOTAStart() { // Perform any necessary tasks before the update begins, such as saving data or closing file handles Serial.println("OTA update starting..."); } void handleOTAProgress(unsigned int progress, unsigned int total) { // Display the progress of the update to the user Serial.printf("Progress: %u%%\r", (progress / (total / 100))); } void handleOTAEnd() { // Perform any necessary tasks after the update is complete, such as restarting the ESP32 Serial.println("\nOTA update complete!"); ESP.restart(); } void setup() { Serial.begin(115200); // Connect to WiFi WiFi.begin(ssid, password); while (WiFi.status() != WL_CONNECTED) { delay(500); Serial.print("."); } // Initialize OTA ArduinoOTA.begin(); ArduinoOTA.setHostname("esp32-ota"); ArduinoOTA.setPassword("password"); // Set up OTA event handlers ArduinoOTA.onStart(handleOTAStart); ArduinoOTA.onProgress(handleOTAProgress); ArduinoOTA.onEnd(handleOTAEnd); } void loop() { ArduinoOTA.handle(); // Your other code goes here }
In this code, the ESP32 connects to the specified WiFi network and then initializes the ArduinoOTA library. It sets the hostname and password for the OTA update and registers event handlers for the start, progress, and end of the update. Finally, the ArduinoOTA.handle() function is called in the main loop of the program to listen for and handle OTA update requests.
Method 2: esp_ghota Library
Update your ESP32 devices in the field directly from GitHub releases by automating your OTA and CI/CD pipeline with GitHub Actions.
Features
- The esp_htps_ota library is used to update firmware images on the ESP32.
- It can also update spiffs/littlefs/fatfs partitions.
- The library uses SemVer to compare versions and will only perform an update if a newer version is available.
- It is compatible with the app rollback and anti-rollback features of the esp-idf bootloader.
- Firmware and partition images can be downloaded from the Github release page.
- The library supports updating multiple devices with different firmware images and includes a sample GitHub Actions workflow for building and releasing images when a new tag is pushed.
- Updates can be triggered manually or at a set interval.
- It uses a streaming JSON parser to reduce the memory usage when processing Github API responses.
- It supports private repositories and GitHub Enterprise using a GitHub API token or personal access token.
- It also sends progress updates through the esp_event_loop.
Detailed descriptions and usage can be found in https://github.com/Fishwaldo/esp_ghota