In this project, we are going to learn to interface a DHT22 sensor with an ESP32 development board and display the readings in the telegram messenger app using the telegram bot API for ESP32.
Hardware required
Libraries Needed
We will be using Arduino IDE for programming the ESP32 board. So, make sure you have the IDE as well as the board package installed on your computer. If you have not set up the Arduino IDE to use with the ESP32 board then follow this tutorial here
We need to install the below 3 libraries in Arduino IDE for reading data from DHTxx module and for communicating with the telegram bot.
Telegram Bot Library
To establish communication with the Telegram bot, we’ll be using the Universal Telegram Bot Library created by Brian Lough that provides an easy interface for Telegram Bot API.
Follow the next steps to install the latest release of the library.
1. Click this link to download the Universal Arduino Telegram Bot library.
2. Go to Sketch > Include Library > Add.ZIP Library...
3. Add the library you’ve just downloaded. And that’s it. The library is installed.
For details about the library, you can check out the Universal Arduino Telegram Bot Library GitHub page.
ArduinoJson Library
We also have to install the ArduinoJson library. Follow the next steps to install the library.
1. Go to Sketch > Include Library > Manage Libraries.
2. Search for “ArduinoJson”.
3. Select the latest version available.
4. Click Install the library.
DHT Sensor Library
Communicating with DHT11, DHT22/AM2302 sensors is difficult. Thank Allah, we don’t have to worry much about this because we are going to use the DHT library from Adafruit which makes things very easy. This library is so good that it runs on both Arduino and ESP architecture.
1. To install the library navigate to the Sketch > Include Library > Manage Libraries
2. Search by typing ‘DHT sensor’. There should be a couple of entries. Look for the DHT sensor library by Adafruit.
3. Click Install the library.
Note
The versions of libraries used while making this tutorial is,
ArduinoJSON – 6.18.5
Telegram Bot – 1.3.0
DHT sensor – 1.4.3
DHT22 Pinout


Circuit
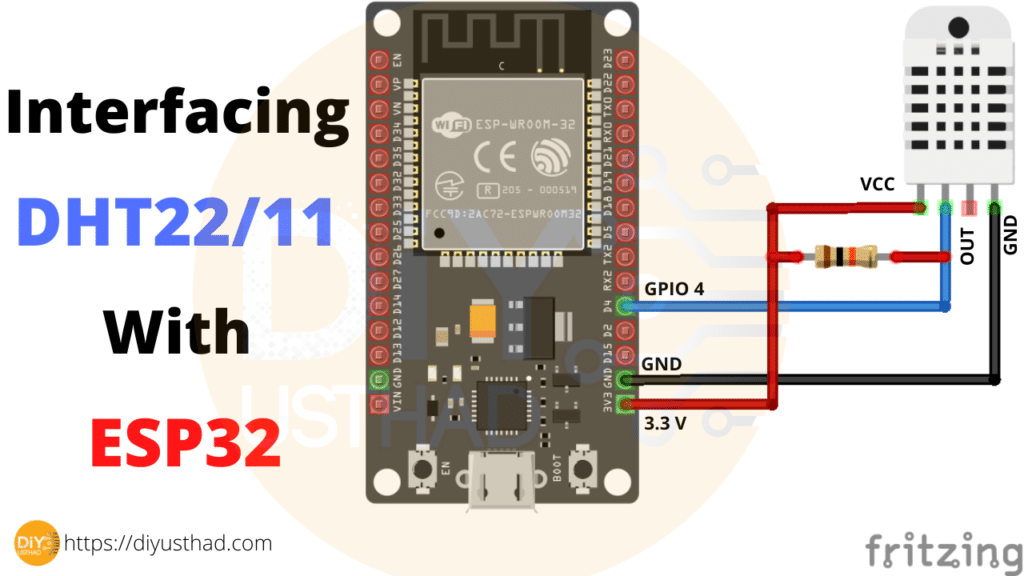
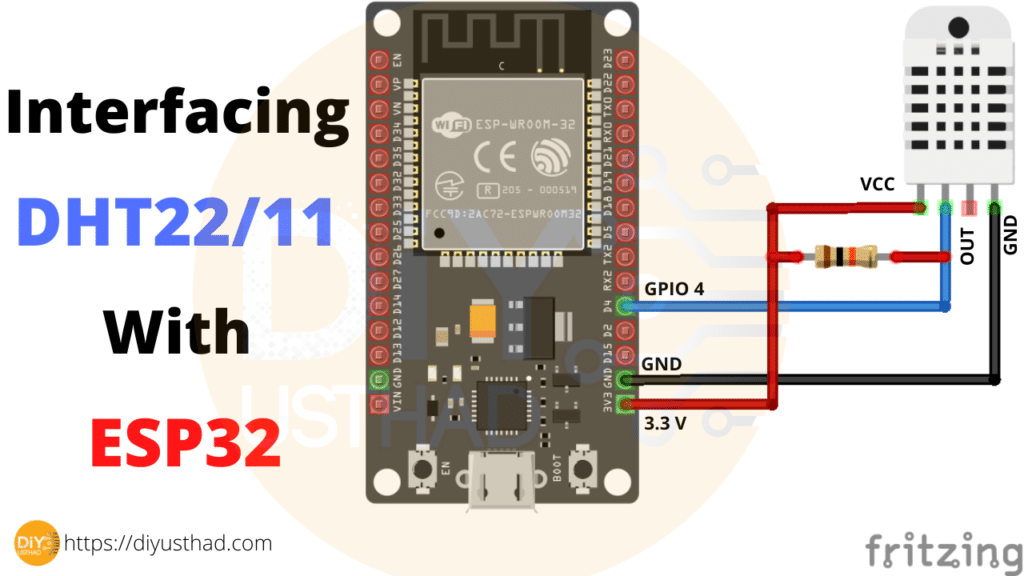
Follow the above circuit diagram and give the connections as follows.
- Connect the GND of DHT22 to GND of ESP32 NodeMCU.
- Connect the VCC of DHT22 to 3.3V of ESP32 NodeMCU.
- Connect the OUT pin of DHT22 to GPIO4 of NodeMCU.
Telegram Bot Creation
We have already explained in detail about bot creation in telegram and obtaining the token in our previous article. Refer from the below link
Code
Add your WiFi credentials, telegram bot token, and chat ID to the code in the mentioned places before uploading.
Choose the current board & port and upload the code.
#include <WiFi.h> #include <WiFiClientSecure.h> #include <UniversalTelegramBot.h> #include "DHT.h" #define DHTPIN 4 // Uncomment whatever type you're using! //#define DHTTYPE DHT11 // DHT 11 #define DHTTYPE DHT22 // DHT 22 (AM2302), AM2321 //#define DHTTYPE DHT21 // DHT 21 (AM2301) // Wifi network station credentials #define WIFI_SSID "YOUR WIFI SSID" #define WIFI_PASSWORD "YOUR WIFI PASSWORD" // Telegram BOT Token (Get from Botfather) #define BOT_TOKEN "YOUR BOT TOKEN" #define CHAT_ID "YOUR CHAT ID" WiFiClientSecure secured_client; UniversalTelegramBot bot(BOT_TOKEN, secured_client); DHT dht(DHTPIN, DHTTYPE); const unsigned long BOT_MTBS = 1000; // mean time between scan messages unsigned long bot_lasttime; // last time messages' scan has been done float temperatureC; float temperatureF; float humidity; void setup() { Serial.begin(9600); Serial.println(F("DHTxx test!")); dht.begin(); // attempt to connect to Wifi network: Serial.print("Connecting to Wifi SSID "); Serial.print(WIFI_SSID); WiFi.begin(WIFI_SSID, WIFI_PASSWORD); secured_client.setCACert(TELEGRAM_CERTIFICATE_ROOT); // Add root certificate for api.telegram.org while (WiFi.status() != WL_CONNECTED) { Serial.print("."); delay(500); } Serial.print("\nWiFi connected. IP address: "); Serial.println(WiFi.localIP()); } void loop() { humidity = dht.readHumidity(); // Read temperature as Celsius (the default) temperatureC = dht.readTemperature(); // Read temperature as Fahrenheit (isFahrenheit = true) temperatureF = dht.readTemperature(true); if (millis() - bot_lasttime > BOT_MTBS) { int numNewMessages = bot.getUpdates(bot.last_message_received + 1); while (numNewMessages) { Serial.println("got response"); handleNewMessages(numNewMessages); numNewMessages = bot.getUpdates(bot.last_message_received + 1); } bot_lasttime = millis(); } } void handleNewMessages(int numNewMessages) { Serial.print("handleNewMessages "); Serial.println(numNewMessages); for (int i = 0; i < numNewMessages; i++) { String chat_id = String(bot.messages[i].chat_id); if (chat_id != CHAT_ID ) { bot.sendMessage(chat_id, "Unauthorized user", ""); } else { String text = bot.messages[i].text; String from_name = bot.messages[i].from_name; if (from_name == "") from_name = "Guest"; if (text == "/tempC") { String msg = "Temperature is "; msg += msg.concat(temperatureC); msg += "C"; bot.sendMessage(chat_id,msg, ""); } if (text == "/tempF") { String msg = "Temperature is "; msg += msg.concat(temperatureF); msg += "F"; bot.sendMessage(chat_id,msg, ""); } if (text == "/humidity") { String msg = "Humidity is "; msg += msg.concat(humidity); msg += "%"; bot.sendMessage(chat_id,msg, ""); } if (text == "/start") { String welcome = "DHTxx sensor readings.\n"; welcome += "/tempC : Temperature in celcius \n"; welcome += "/tempF : Temperature in faranthit\n"; welcome += "/humidity : Humidity\n"; bot.sendMessage(chat_id, welcome, "Markdown"); } } } }