In this project, we are going to build an ESP32-based Islamic Prayer time display. Instead of calculating the prayer time using coordinates, we are going to fetch the prayer time data from the internet and display it on an LCD display.
Video
Things Needed
Components | AliExpress![]() ![]() | Amazon![]() ![]() |
---|---|---|
ESP32 (NodeMCU) | ![]() ![]() | ![]() ![]() |
LCD 1602 | ![]() ![]() | ![]() ![]() to buy i2c converter) |
LCD i2c convertor module | ![]() ![]() | ![]() ![]() |
Circuit
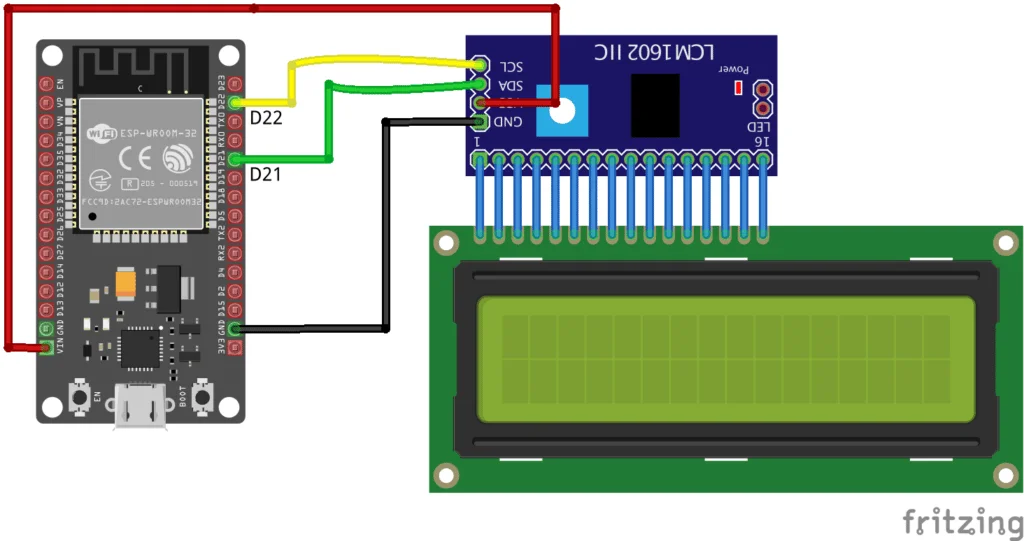
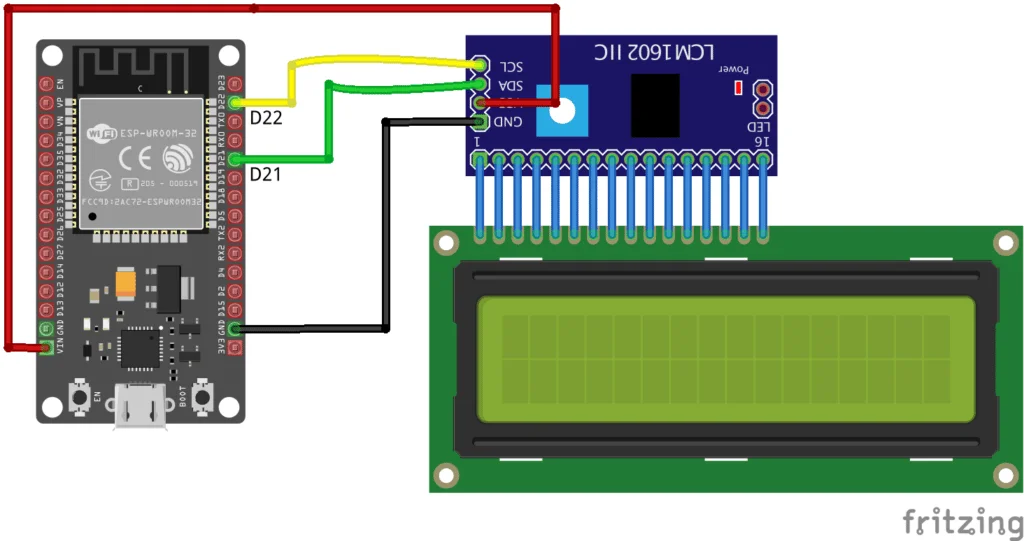
ESP32 (NodeMCU) | LCD 16×02 (with I2C) |
---|---|
GPIO 21 (SDA) | SDA |
GPIO 22 (SCL) | SCL |
GND | GND |
VIN | VCC |
Prayer Time API
To fetch the prayer time we are going to use the API provided by https://aladhan.com/. They are providing a lot of parameters to fetch different types of details. In our case, we need to fetch timings by city(read more).
For example, if we need time for Dubai then the API request will look like this,
API Request: http://api.aladhan.com/v1/timingsByCity?city=Dubai&country=United Arab Emirates&method=4
To check whether the API request URL is correct you can simply visit it from your browser and it should return you with the JSON data of the prayer time. I’m attaching the screenshot of the above URL below
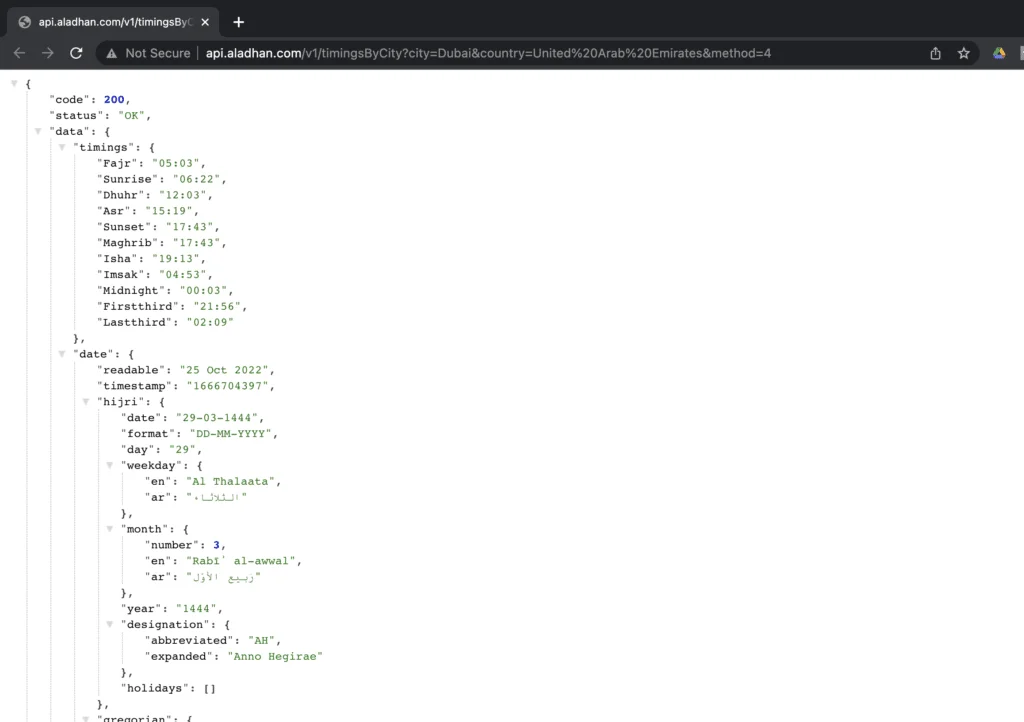
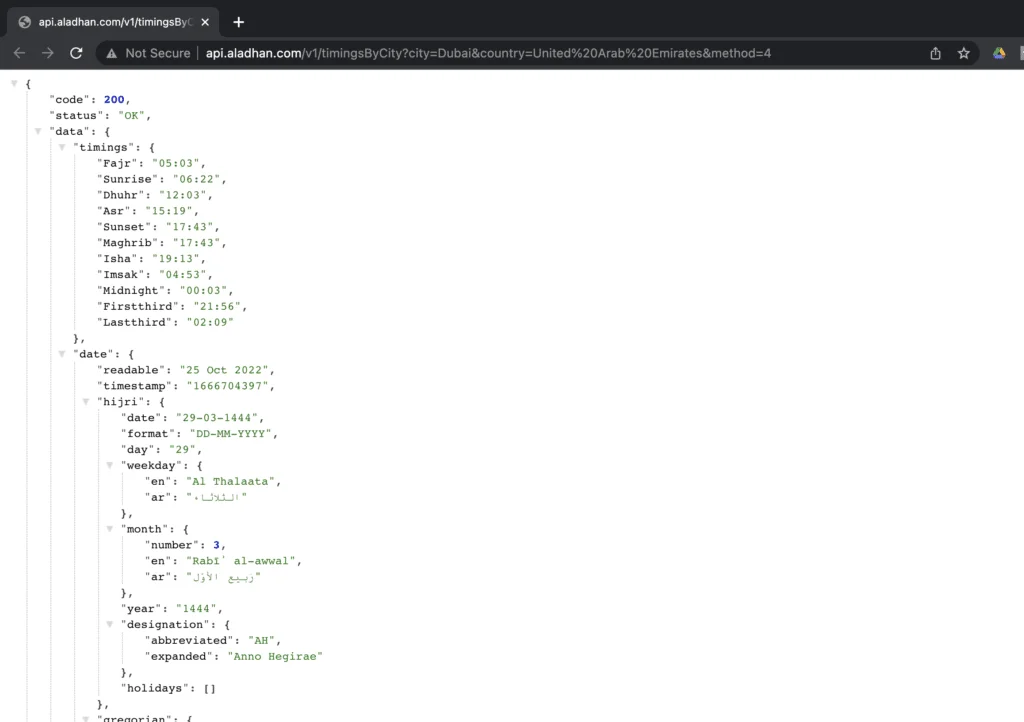
Libraries Used
Libraries | GitHub |
---|---|
LiquidCrystal_I2C Library by Marco Schwartz | ![]() ![]() |
ArduinoJson Library | ![]() ![]() |
Installing the LiquidCrystal_I2C Library
1. Click here to download the LiquidCrystal_I2C library. You should have a .zip file in your Downloads
2. Unzip the file and Rename the folder from LiquidCrystal_I2C-master to LiquidCrystal_I2C
3. Then move the LiquidCrystal_I2C folder to your Arduino IDE installation libraries folder
4. Now, re-open your Arduino IDE
ArduinoJson Library
We also have to install the ArduinoJson library. Follow the next steps to install the library.
1. Go to Sketch > Include Library > Manage Libraries.
2. Search for “ArduinoJson”.
3. Select the latest version available.
4. Install the library.
Code
In the code below you have to change the following things before uploading.
- Write your WiFi SSID and Password where it’s mentioned.
- Change the API request URL with the URL you obtained from the aladhan website.
- Dont forget to replace https with http because this program cannot handle HTTPS
//program by https://diyusthad.com #include <SPI.h> #include <Wire.h> #include <WiFi.h> #include <HTTPClient.h> #include <ArduinoJson.h> #include <LiquidCrystal_I2C.h> const char* ssid = "YOUR_SSID"; const char* password = "YOUR_WIFI_PASSWORD"; int lcdColumns = 16; int lcdRows = 4; LiquidCrystal_I2C lcd(0x27, lcdColumns, lcdRows); const char* data_timings_Fajr ; const char* data_timings_Sunrise ; const char* data_timings_Dhuhr ; const char* data_timings_Asr ; const char* data_timings_Sunset ; const char* data_timings_Maghrib ; const char* data_timings_Isha ; const char* data_timings_Imsak ; const char* data_timings_Midnight ; void setup() { Serial.begin(115200); WiFi.begin(ssid, password); Serial.println("Connecting"); while (WiFi.status() != WL_CONNECTED) { delay(500); Serial.print("."); } Serial.println(""); Serial.print("Connected to WiFi network with IP Address: "); Serial.println(WiFi.localIP()); lcd.init(); // turn on LCD backlight lcd.setBacklight(10); fetchData(); } void loop() { lcd.clear(); lcd.setCursor(0, 0); lcd.print("Fajr : "); lcd.print(data_timings_Fajr); lcd.setCursor(0, 1); lcd.print("Dhuhr : "); lcd.print(data_timings_Dhuhr); delay(2000); lcd.clear(); lcd.setCursor(0, 0); lcd.print("Asr : "); lcd.print(data_timings_Asr); lcd.setCursor(0, 1); lcd.print("Maghrib : "); lcd.print(data_timings_Maghrib); delay(2000); lcd.clear(); lcd.setCursor(0, 0); lcd.print("Isha : "); lcd.print(data_timings_Isha); delay(2000); } void fetchData() { if (WiFi.status() == WL_CONNECTED) { HTTPClient http; Serial.print("[HTTP] begin...\n"); if (http.begin("http://api.aladhan.com/v1/timingsByCity?city=Dubai&country=United%20Arab%20Emirates&method=4")) { // API Request Serial.print("[HTTP] GET...\n"); // start connection and send HTTP header int httpCode = http.GET(); // httpCode will be negative on error if (httpCode > 0) { // HTTP header has been send and Server response header has been handled Serial.printf("[HTTP] GET... code: %d\n", httpCode); // file found at server if (httpCode == HTTP_CODE_OK || httpCode == HTTP_CODE_MOVED_PERMANENTLY) { String payload = http.getString(); DynamicJsonDocument doc(2048); deserializeJson(doc, payload); JsonObject data = doc["data"]; JsonObject data_timings = data["timings"]; data_timings = data["timings"]; data_timings_Fajr = data_timings["Fajr"]; // "05:13" data_timings_Sunrise = data_timings["Sunrise"]; // "06:31" data_timings_Dhuhr = data_timings["Dhuhr"]; // "12:29" data_timings_Asr = data_timings["Asr"]; // "15:52" data_timings_Sunset = data_timings["Sunset"]; // "18:26" data_timings_Maghrib = data_timings["Maghrib"]; // "18:26" data_timings_Isha = data_timings["Isha"]; // "19:56" data_timings_Imsak = data_timings["Imsak"]; // "05:03" data_timings_Midnight = data_timings["Midnight"]; // "00:29" //Serial.println("fajr time:"); //Serial.println(data_timings_Fajr); //Serial.println(data_timings_Dhuhr); //Serial.println(data_timings_Asr); //Serial.println(data_timings_Maghrib); //Serial.println(data_timings_Isha); } } else { Serial.printf("[HTTP] GET... failed, error: %s\n", http.errorToString(httpCode).c_str()); } http.end(); } else { Serial.printf("[HTTP} Unable to connect\n"); } } }
Hello there, im having a problem here, when i run this Code without LCD I2C, (so i just print it in the serial monitor..) and it says failed, error. what is that mean, i try to use ur API , and also didnt give me the prayer tines.. Im using the API, but my API but unlike yours.. mine was printed all horizontally please help me figure this out thankyouu